http://www.appcoda.com/model-view-controller-delete-table-row-from-uitableview/
How To Delete a Row from UITableView
I hope you have a better understanding about Model-View-Controller. Now let’s move onto the coding part and see how we can delete a row from UITableView. To make thing simple, I’ll use the plain version of Simple Table app as an example.If you thoroughly understand the MVC model, you probably have some ideas how to implement row deletion. There are three main things we need to do:
1. Write code to switch to edit mode for row deletion
2. Delete the corresponding table data from the model
3. Reload the table view in order to reflect the change of table data
1. Write code to switch to edit mode for row deletion
In iOS app, user normally swipes across a row to initiate the delete button. Recalled that we have adopted the UITableViewDataSource protocol, if you refer to the API doc, there is a method named tableView:commitEditingStyle:forRowAtIndexPath. When user swipes across a row, the table view will check to see if the method has been implemented. If the method is found, the table view will automatically show the “Delete” button.Simply add the following code to your table view app and run your app:
1
2 3 4 |
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{ } |
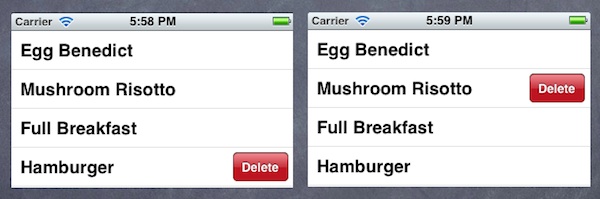
Swipe to Delete a Table Row
2. Delete the corresponding table data from the model
The next thing is to add code to the method and remove the actual table data. Like other table view methods, it passes the indexPath as parameter that tells you the row number for the deletion. So you can make use of this information and remove the corresponding element from the data array.In the original code of Simple Table App, we use NSArray to store the table data (which is the model). The problem of NSArray is it’s non-editable. That is, you can’t add/remove its content once the array is initialized. Alternatively, we’ll change the NSArray to NSMutableArray, which adds insertion and deletion operations:
1
2 3 4 5 6 7 8 9 10 11 |
@implementation SimpleTableViewController
{ NSMutableArray *tableData; } - (void)viewDidLoad { [super viewDidLoad]; // Initialize table data tableData = [NSMutableArray arrayWithObjects:@"Egg Benedict", @"Mushroom Risotto", @"Full Breakfast", @"Hamburger", @"Ham and Egg Sandwich", @"Creme Brelee", @"White Chocolate Donut", @"Starbucks Coffee", @"Vegetable Curry", @"Instant Noodle with Egg", @"Noodle with BBQ Pork", @"Japanese Noodle with Pork", @"Green Tea", @"Thai Shrimp Cake", @"Angry Birds Cake", @"Ham and Cheese Panini", nil]; } |
1
2 3 4 5 |
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{ // Remove the row from data model [tableData removeObjectAtIndex:indexPath.row]; } |
It’s not a bug. The app does delete the item from the array. The reason why the deleted item still appears is the view hasn’t been refreshed to reflect the update of the data model.
3. Reload the table view
Therefore, once the underlying data is removed, we need to invoke “reloadData” method to request the table View to refresh. Here is the updated code:
1
2 3 4 5 6 7 8 |
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{ // Remove the row from data model [tableData removeObjectAtIndex:indexPath.row]; // Request table view to reload [tableView reloadData]; } |
Test Your App and Delete a Row
Try to run your app again and swipe to delete a row. You should be able to delete it.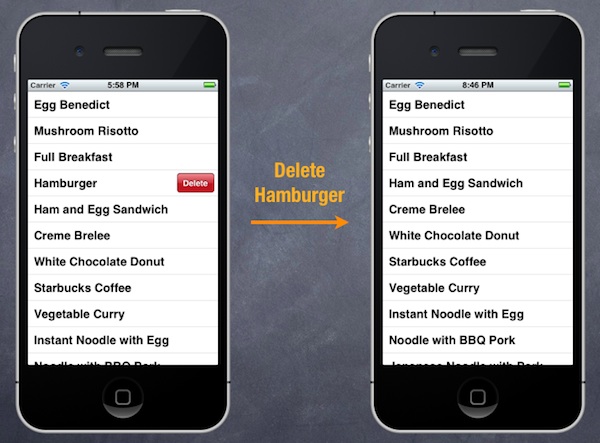
Delete a Table Row in Simple Table App
No comments:
Post a Comment